Running Prettier for specific Git branch
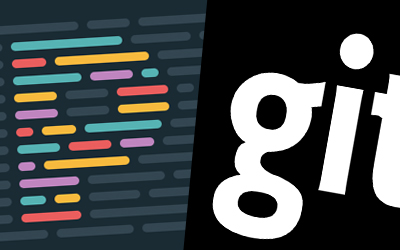
I'm working on a project in my own git branch. At the end of my working day I want to do one thing - run Prettier so my code is nicely formatted before I submit the PR for code review. Of course I know about the VSCode Prettier extension but right now my editor is kinda broken and I don't want to mess with it. I'm still on my branch and I run Prettier from the terminal. All good but the amount of changes that happened is so big that my 50+ loc updates are like a needle in a haystack. It is like that because apparently there are already malformatted files in the project. It is definitely not possible to understand what I did because of so much noise. So ... I want to run Prettier but only on the files that I touched in that particular branch.
Git to the rescue! I had to compare my branch to master
, find out what are the changed files and run Prettier only for them. I decided to write a small shell script that will do this job for me. I will use it now and in the future.
#!/bin/sh
BASEDIR="<path to my project dir>"
files=$(git diff --name-status master);
while read -r file; do
mode=$(echo "$file" | awk '{print $1}')
filePath=$(echo "$file" | awk '{print $2}')
if [ "$mode" = "M" ] || [ "$mode" = "A" ] || [ "$mode" = "AM" ]
then
npx prettier --write $filePath
fi
done <<< "$files"
Let's go over it line by line.
files=$(git diff --name-status master);
- runsgit diff
comparing the current branch tomaster
.--name-status
is giving us a nice two column table. The first one is what type of change we did and the second one the file path.while read -r file; do
- we loop over the table rows.mode=$(echo "$file" | awk '{print $1}')
- this line gives us the content of the first column.filePath=$(echo "$file" | awk '{print $2}')
- similarly to the line above this one gives us the value of the second column (the file path).if [ "$mode" = "M" ] || [ "$mode" = "A" ] || [ "$mode" = "AM" ]
- we check if the file is modified but not deleted. Prettier will scream if we run it against a file that doesn't exists.npx prettier --write $filePath
- at the end we format the file.
I hope it's useful. 😎